Server to Server (v2)
The Transactions API allows you create and manage payments on your integration
- Encrypt your Card (Card Number, Expiry Date, CVV and PIN) with
- Pass your secret key as the Bearer token in Authorization Header.
- Encrypt the whole payout using your pub_key and Pass your HMAC Signature as Encryption Header. (Required only in Payout and Bills Payment)
Card Encryption (v2)
Card Encryption: perform aes-256-cbc encryption on your card payload using your pub_key and reference
To generate a test card encryption, kindly use our test encryption endpoint below
Endpoint: /test/encryption
Method: POST
Header
Param | Type | Required? | Decription |
---|---|---|---|
authorization | string | Yes | Set value to Bearer SECRET_KEY |
content-type | string | Yes | Set value to application/json |
Card Encryption Payload
{
"data" :{
"number": "5123450000000008",
"expiryMonth": "10",
"expiryYear": "22",
"cvv" : "100",
"pin" : "1234" // (optional - only required for local cards)
},
"reference": "1253627873656276350"
}
Card Encryption Output
{
"card" :"83fa6763bb31bae36a74f787ab814514aeede91fcdb311fd67609b414c5e5ea2751a47870c8717e71bcbc9c33287a3d6af9ffae8e2edbf2de1e2694384d699b020d31492637eef60d7a63f303798363a"
}
Initialize Transaction (S2S v2)
Initialize a transaction from your backend.
You are required to parse your card encryption output as card parameter in your Transaction Initialize payload.
You are expected to perform HMAC-SHA-512 encryption on your Transaction Initialize payload using your secret_key and parse it as Encryption in your Header. The parameters of this payload to encrypt must be in Alphabetical order.
Endpoint: /transaction/initialize
Method: POST
Header
Param | Type | Required? | Decription |
---|---|---|---|
authorization | string | Yes | Set value to Bearer SECRET_KEY |
encryption | string | Yes | Set value to your Signature_HMAC-SHA-512 output |
content-type | string | Yes | Set value to application/json |
Sample Post
curl https://api.budpay.com/api/s2s/v2/transaction/initialize
-H "Authorization: Bearer YOUR_SECRET_KEY"
-H "Encryption: Signature_HMAC-SHA-512"
-H "Content-Type: application/json"
-d '{ "amount": "100", "card" :"83fa6763bb31bae36a74f787ab814514aeede91fcdb311fd67609b414c5e5ea2751a47870c8717e71bcbc9c33287a3d6af9ffae8e2edbf2de1e2694384d699b020d31492637eef60d7a63f303798363a", "currency": "USD", "email": "test@email.com", "reference": "1253627873656276350" }'
-X POST
3DS2 Sample Response
{
"status": true,
"message": "Proceed authentication 3DS2",
"data": "<div id=\"initiate3dsSimpleRedirect\" xmlns=\"http://www.w3.org/1999/html\"> <iframe id=\"methodFrame\" name=\"methodFrame\" height=\"100\" width=\"200\" > </iframe> <form id =\"initiate3dsSimpleRedirectForm\" method=\"POST\" action=\"https://secure-acs2ui-b1.wibmo.com/v1/acs/services/threeDSMethod/8528?cardType=M\" target=\"methodFrame\"> <input type=\"hidden\" name=\"threeDSMethodData\" value=\"eyJ0aHJlZURTTWV0aG9kTm90aWZpY2F0aW9uVVJMIjoiaHR0cHM6Ly9ldS5nYXRld2F5Lm1hc3RlcmNhcmQuY29tL2NhbGxiYWNrSW50ZXJmYWNlL2dhdGV3YXkvOGFkZGRiNzE1MTQwMjk2OWMxMmI1Y2QzNjBhOTdmYTdiZTE1ZWU1NWZiZDEwZTE5MmI3ZDIwNDcxNmQxYTVlNSIsInRocmVlRFNTZXJ2ZXJUcmFuc0lEIjoiZjgxNGM3YWMtMmRiOS00Nzg1LThhMjYtYjA2NjZlYTBjMGVkIn0=\" /> </form> <script id=\"initiate-authentication-script\"> var e=document.getElementById(\"initiate3dsSimpleRedirectForm\"); if (e) { e.submit(); if (e.parentNode !== null) { e.parentNode.removeChild(e); } } </script> </div>",
"_links": {
"url": "http://api.budpay.com/api/mastercard-payment/3ds2auth",
"method": "POST",
"payload": [
"ref"
]
}
}
You are required to open the 3DS2 Response data html in a hidden page to run in background for 20 seconds and then hit the _links endpoint in the response to proceed. After that you will get the Final Response.
Note: You will receive 3DS2 response for cards that support 3DS2. If not, you will get the Final Response instead.
Final Sample Response
{
"status": "success",
"message": "Approved or Captured"
}
NOTE: Before giving value to your customers, kindly confirm if the “STATUS is SUCCESSFUL, and the MESSAGE is either APPROVED or CAPTURED.”
Parameters
Param | Type | Required? | Decription |
---|---|---|---|
string | Yes | Customer email address | |
amount | string | Yes | Amount you are debiting customer. Do not pass this if creating subscriptions. |
card | string | Yes | Encrypted Card output from card encryption. |
currency | string | no | Currency charge should be performed in. Allowed values are: NGN, USD or GBP It defaults to your integration currency. Default currency set to NGN when currency parameter is not set |
reference | string | Yes | Unique case sensitive transaction reference. Only -,_,., =and alphanumeric characters allowed. |
3DS2 Flow Diagram
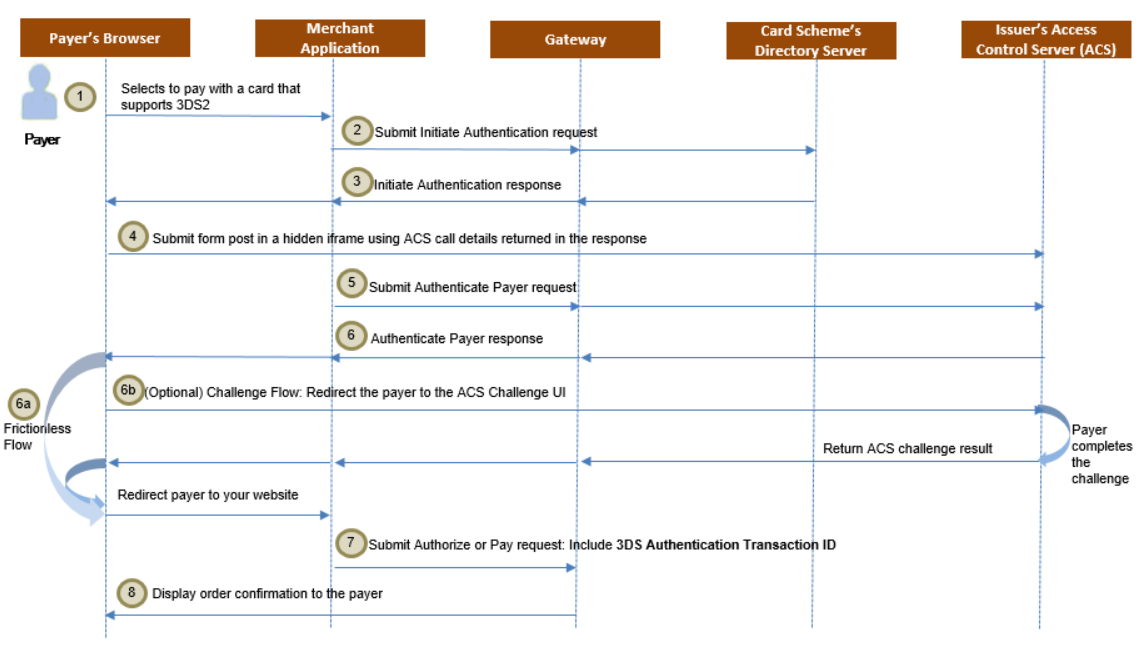